This weekend I was coming home from the meeting of theLSST Dark Energy Science Collaboration,and found myself with a few extra hours in the airport.I started passing the time by poking around on the imgurgallery, and saw a couple animated gifs based onone of my all-time favorite games, Super Mario Bros.It got me wondering: could I use matplotlib's animation tools to create thesesorts of gifs in Python? Over a few beers at an SFO bar, I started to tryto figure it out. To spoil the punchline a bit, I managed to do it, and theresult looks like this:
Super Mario Bros 64 Seeing ROM hacks for Mario games, or indeed any Nintendo classic is hardly unusual. For those unaware of the term, this is essentially where existing assets from a game are. Super Mario Bros. 1X: Added: 2018-08-26 05:43:15 PM: Authors: Koyuki: Demo: No Featured: No Length: 66 exit(s) Type: Standard: Normal Description: This is a hack that is largely inspired by Super Mario All-Stars and contains many references to the game. Super Mario Bros 1.5 v1.0 (SMB1 Hack). 32.0 KB (4) This rom is not available for download. It is protected by the ESA. Now and enjoy: Ad-free.
This animation was created entirely in Python and matplotlib, by scraping theimage data directly from the Super Mario Bros. ROM. Below I'll explain howI managed to do it.
Scraping the Pixel Data
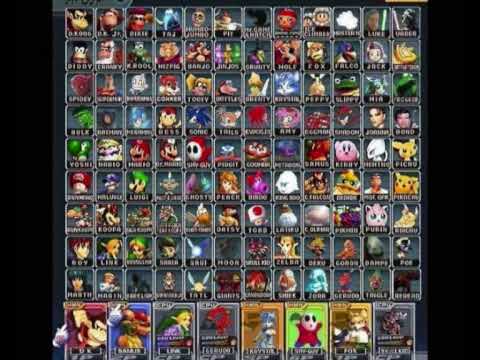
Clearly, the first requirement for this pursuitis to get the pixel data used to construct themario graphics. My first thought was to do something sophisticated likedictionary learning on a collection of screen-shots from the gameto build up a library of thumbnails. That would be an interesting pursuitin itself, but it turns out it's much more straightforward to directlyscrape the graphics from the source.
It's possible to find digital copies of mostNintendo Entertainment System (NES) games online.These are known as ROMs, and can be played using one ofseveral NES emulators available for various operating systems.I'm not sure about the legality of thesedigital game copies, so I won't provide a link to them here. But the internetbeing what it is, you can search Google for some variation of 'Super MarioROM' and pretty easily find a copy to download.
One interesting aspect of ROMs for the original NES is thatthey use raw byte-strings to store 2-bit (i.e. 4-color), 8x8 thumbnails fromwhich all of the game's graphics are built.The collection of these byte-stringsare known as the 'pattern table' for the game, and there is generally aseparate pattern table for foreground and background images.In the case of NES games, there are256 foreground and 256 background tiles, which can be extracted directly fromthe ROMs if you know where to look (incidentally, this is one of the thingsthat made the NES an '8-bit' system. 2^8 = 256, so eight bits are requiredto specify any single tile from the table).
Extracting Raw Bits from a File
If you're able to obtain a copy of the ROM, the first step to getting at thegraphics is to extract the raw bit information.This can be done easily in Python using numpy.unpackbits
and numpy.frombuffer
or numpy.fromfile
.Additionally, the ROMs are generally stored usingzip compression. The uncompressed data can be extracted using Python'sbuilt-in zipfile
module. Combining all of this, we extract the raw filebits using a function like the following:
This function checks whether the file is compressed using zip, and extractsthe raw bit information in the appropriate way.
Assembling the Pattern Tables
The thumbnails which contain the game's graphics patterns are not at any setlocation within the file. The location is specified within the assemblycode that comprises the program, but for our purposesit's much simpler to just visualizethe data and find it by-eye. To accomplish this,I wrote a Python script(download it here)based on the above data extraction codewhich uses matplotlib to interactively display the contents of the file.Each thumbnail is composed from 128 bits:two 64-bit chunks each representing an 8x8 image with one bit per pixel.Stacking the two results in two bits per pixel, which are able torepresent four colors within each thumbnail.The first few hundred chunks are difficult to interpret by-eye. They appearsimilar to a 2D bar code: in this case the 'bar code' represents pieces of theassembly code which store the Super Mario Bros. program.
Scrolling down toward the end of the file, however, we can quickly recognizethe thumbnails which make up the game's graphics:
This first pattern table contains all the foreground graphics for the game.Looking closely, the first few thumbnailsare clearly recognizable as pieces of Mario's head and body.Going on we see pieces of various enemies in the game, as well as the iconicmushrooms and fire-flowers.
The second pattern table contains all the background graphics for the game.Along with numbers and text, this contains the pieces which make up mario'sworld: bricks, blocks, clouds, bushes, and coins.Though all of the above tiles are shown in grayscale, we can add color bysimply changing the matplotlib Colormap, as we'll see below.
Combining Thumbnails and Adding Color
Examining the pattern tables above, we can see that big Mario is made up ofeight pattern tiles stitched together, while small Mario is made up of four.With a bit of trial and error, we can create each of the full frames andadd color to make them look more authentic. Below are all of the frames usedto animate Mario's motion throughout the game:
Similarly, we can use the thumbnails to construct some of the otherfamiliar graphics from the game, including the goombas, koopa troopas,beetle baileys, mushrooms, fire flowers, and more.
The Python code to extract, assemble, and plot these images can be downloadedhere.
Animating Mario
With all of this in place, creating an animation of Mario is relatively easy.Using matplotlib's animation tools (described in aprevious post), all ittakes is to decide on the content of each frame, and stitch the frames togetherusing matplotlib's animation toolkit. Putting together big Mario with somescenery and a few of his friends, we can create a cleanly looping animated gif.
The code used to generate this animation is shown below. We use the sameNESGraphics
class used to draw the frames above, and stitch them togetherwith a custom class that streamlines the building-up of the frames.By uncommenting the line near the bottom, the result will be saved as ananimated GIF using the ImageMagick animation writer that Irecently contributedto matplotlib. The ImageMatick plugin has not yet made it into areleased matplotlib version, so using the save command below willrequire installing the development version of matplotlib, available fordownload on github.
The result looks like this:
Pretty good! With a bit more work, it wouldbe relatively straightforward to use the above code to do some moresophisticated animations: perhaps recreate a fulllevel from the original Super Mario Bros, or even design your own customlevel. You might think about taking the extra step and trying to make Mario'smovements interactive. This could be a lot of fun, but probably very difficultto do well within matplotlib.For tackling an interactive mario in Python, another framework such asTkinter orpygame might be a better choice.
Pdf epub drm removal for mac. I hope you enjoyed this one as much as I did -- happy coding!
I am pretty sure the whole time I am writing this Super Mario Bros review I will have a big goofy smile on my face. I first played the original Mario game back in the 80s on my uncle's NES. That Christmas I knew what I wanted Santa to bring me and I was not disappointed. This is a game I (and most probably everyone reading this) know very well. I decided to play through it again for this review to see just how well it holds up.
Where The Heck Is The Princess?
No one is going to accuse the original or actually any of the Super Mario Bros games of being deep when it comes to the story. You are playing as Super Mario and you need to save the Princess and put a stop to King Koopa from causing trouble in the Mushroom Kingdom. The fact of the matter is, you do not care about the story in a Mario game, but at the same time, you always want to put King Koopa in his place.
Where It All Began
It is crazy that we are not all that far off from this game being 40 years old as I write this. Bwanadik 4.1.0 10.11.5 for mac. I say that because the original Super Mario Bros has aged like a fine wine. Yes, Super Mario Bros 3, Super Mario World and the various New Super Mario Bros games have taken this formula and improved on it in every way you could imagine. The fact of the matter is, Super Mario Bros is still a joy to play.
What I think this game does very, very well is make it so that pretty much any gamer, no matter their skill level can get through the first world. After this, the game ramps up the difficulty and requires a great deal of skill to get to the end. Even the final castle level which has to be completed in a specific way was a big deal back when this game was first released.
Classic
Many games like to think they are classic, but I do not think you get much more classic than Super Mario Bros. The visuals are very primitive, but man there is so much personality here. The easiest way to prove this is the sheer amount of merchandise that is in game stores, supermarkets and so on that is all based on the original Mario Bros design. This shows just how timeless this version of Mario really is. It is not just people in their 30s and 40s, but kids as well have a real love for this original look of Mario.
Super Mario Bros 2 Player Hack Rom Download
I would not say that the original Super Mario Bros is my favorite Mario game. However, this still holds up and it is a fun game to play. This is one of those games that I personally feel every gamer needs to have played. It is a fun experience and one that is far more challenging than many people realize.
Final Score: 9/10
Pros:
- The Mario theme never gets old
- Classic Mario has tons of charm
- A true platforming classic
- The game is way more challenging than you would think
- Great for people of all ages
Super Mario Bros Ds Rom Hacks Download
Cons:
Newer Super Mario Bros Ds Rom Hack Download
- The following Mario games do improve on the formula
- I must admit I prefer the GameBoy Color version